How to Extract an Image from MP4 Using JavaScript
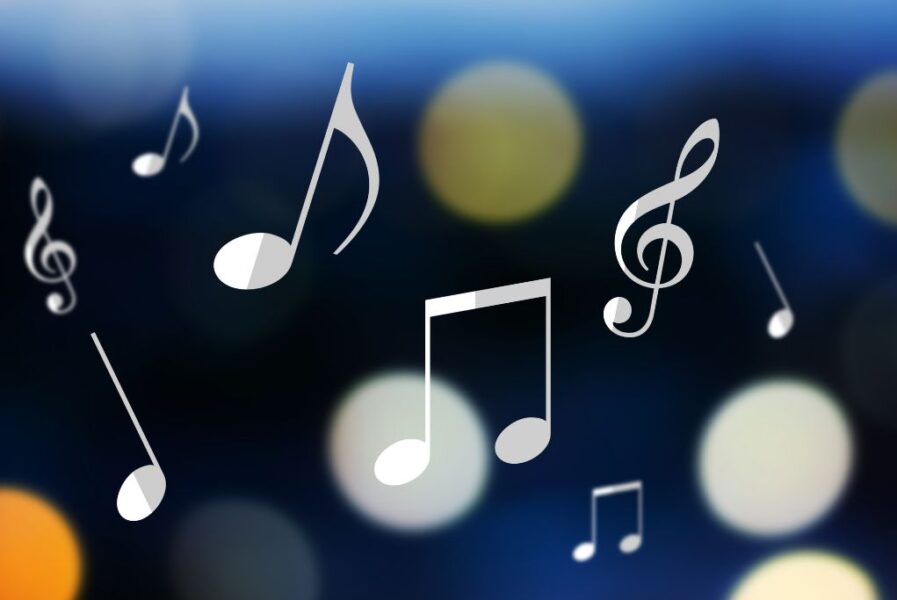
This guide explains how to extract an image from MP4 using JavaScript, HTML5 video, canvas elements, and the drawImage method in JavaScript.
This technique allows you to play the video, set a time, and capture video frames as images on the canvas. After capturing the frame, you can save it as a downloaded image.
It’s super straight forward and we even provide a full working code example you can copy and use, so keep reading.
How to Extract an Image from MP4 Using JavaScript
Here’s the complete process:
- Import the MP4 video using an
<input>
file element or as the video source - Use the
<video>
element to play the video hidden (offscreen) - Look up a specific time in the video using the
currentTime
property - Create the image of the video frame with
<canvas>
element using thedrawImage()
method - Copy the image from canvas and convert it to a data URL using
toDataURL()
if you want to download or display it
HTML and JavaScript Code
Here’s the complete implementation:
HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Extract Image from MP4</title>
</head>
<body>
<h1>Extract Image from MP4 Video</h1>
<!-- File Input -->
<input type="file" id="videoInput" accept="video/mp4" />
<br /><br />
<!-- Canvas to Position the Extracted Frame -->
<canvas id="videoCanvas" style="border: 1px solid #ddd; display: none;"></canvas>
<br />
<!-- Image Output -->
<img id="imageOutput" alt="This Extracted Frame Will Be Displayed Here" style="max-width: 100%;"/>
<script>
// Select elements
const videoInput = document.getElementById('videoInput');
const videoCanvas = document.getElementById('videoCanvas');
const imageOutput = document.getElementById('imageOutput');
const canvasContext = videoCanvas.getContext('2d');
// Add a video element (hidden)
const videoElement = document.createElement('video');
videoElement.crossOrigin = "anonymous"; // For CORS supported videos
videoElement.style.display = "none"; // Hide it
videoElement.muted = true; // Autoplay videos in mute mode
// File input event listener
videoInput.addEventListener('change', function(event) {
const file = event.target.files[0]; // Get the current video file
if (file) {
const fileURL = URL.createObjectURL(file); // Get the URL of the video
videoElement.src = fileURL; // Set video source for element
videoElement.load(); // Load the video
videoElement.addEventListener('loadeddata', function() {
captureFrameAtTime(2); // Pull frame from 2 seconds (example)
});
}
});
// Function to capture video frame at specific time
function captureFrameAtTime(timeInSeconds) {
videoElement.currentTime = timeInSeconds;
videoElement.addEventListener('seeked', function onSeeked() {
// Set canvas size to match video
videoCanvas.width = videoElement.videoWidth;
videoCanvas.height = videoElement.videoHeight;
// Draw the video frame in the current state on canvas
canvasContext.drawImage(videoElement, 0, 0, videoCanvas.width, videoCanvas.height);
// Transform canvas object into data URL (image)
const imageDataURL = videoCanvas.toDataURL('image/png');
// Display the extracted image
imageOutput.src = imageDataURL;
// Clean up: remove the event listener
videoElement.removeEventListener('seeked', onSeeked);
});
}
</script>
</body>
</html>
How the Code Works
File Input
- An
<input>
element is used to accept an MP4 video file - The file input triggers the frame extraction process
Video Loading
- An invisible
<video>
element is dynamically created - The uploaded video is loaded using
URL.createObjectURL()
- The video loads in the background, hidden from view
Seek a Specific Time
- The video’s
currentTime
the attribute is set to seek a specific timestamp - The system waits for the video to reach the specified time
Extract and Draw Image
- When the video seeks the specified time, the ‘seeked‘ event triggers
- The canvas dimensions are set to match the video dimensions exactly
drawImage()
captures the current video frame and draws it to the canvas- The frame is captured at full resolution
Export Image
toDataURL()
converts the canvas content into a PNG image- The resulting image data is displayed in an
<img>
tag - The image can be saved or downloaded
4. Output
After uploading an MP4 video, the script:
- Extracts a frame (default is at 2 seconds)
- Outputs it as an image
- Displays the image on the page
- Allows saving via right-click or programmatic download
5. Key Notes
Cross-Origin Videos
- When pulling videos from URLs, ensure proper CORS headers are set
- Use
crossOrigin="anonymous"
for CORS-enabled videos - Security warnings may appear when using
toDataURL()
without proper CORS settings
Timing Precision
- Frame accuracy depends on video encoding and browser behavior
- Some timestamps may not align perfectly with frame boundaries
- Browser implementation affects the precision of frame extraction
Performance Considerations
- Extracting multiple frames requires careful performance optimization
- Consider buffering frames for smoother operation
- Large videos may require additional processing time
6. Optional Enhancements
User Interface Improvements
- Add controls for users to set custom extraction timestamps
- Implement a preview system for frame selection
- Add a progress indicator for longer videos
Export Options
- Add a dedicated download button for extracted frames
- Support multiple image formats (PNG, JPEG, WebP)
- Include quality settings for exported images
Advanced Features
- Enable extraction of multiple frames at specified intervals
- Create thumbnail sequences automatically
- Add video playback controls for precise frame selection
- Implement batch processing capabilities
Browser Compatibility
This implementation works in modern browsers that support the following:
- HTML5 Video API
- Canvas API
- File API
- Blob URLs
Specifically supported in:
- Google Chrome 40+
- Mozilla Firefox 42+
- Safari 10+
- Microsoft Edge 79+
- Opera 27+
Make sure to test in your target browsers and add the correct fallbacks if necessary.
If this article helped you, make sure you check out how to Play a MP3 File in JavaScript!